Tracing mechanism in Windows Communication Foundation is based on the classes that resides in System.Diagnostic namespace. Important classes are Trace, TraceSource and TraceListener. For better understanding, i'm gonna explain to you step by step approach in this WCF Tutorial.
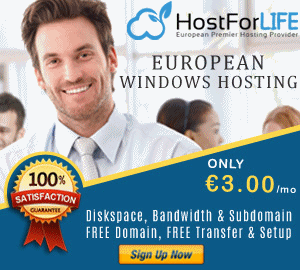
Follow these steps to enable tracing in WCF:
Step 1: Configuring WCF to emit tracing information/Define Trace Source
You will have the following options:
- System.ServiceModel
- System.ServiceModel.MessageLogging
- System.ServiceModel.IdentityModel
- System.ServiceModel.Activation
- System.Runtime.Serialization
- System.IO.Log
- Cardspace
In configuration file, you need define a source to enable this configuration as follows:
<source name=”System.ServiceModel.MessageLogging”>
Step 2: Setting Tracing Level
We have the following available options:
- Off
- Critical
- Error
- Warning
- Information
- Verbose
- ActivityTracing
- All
And we need to set this tracing level to available options other than default OFF.
In configuration file, we can choose above values for switchValue attribute as follows:
<source name=”System.ServiceModel.MessageLogging”
switchValue=”Information”>
Step 3: Configuring a Trace Listener
For configuring a trace listener, we will add following to config file:
<listeners>
<add name=”messages”
type=”System.Diagnostics.XmlWriterTraceListener”
initializeData=”d:logsmessages.svclog” />
</listeners>
Step 4: Enabling Message Logging
Follow this code below to enable message logging:
<system.serviceModel>
<diagnostics>
<messageLogging
logEntireMessage=”true”
logMalformedMessages=”false”
logMessagesAtServiceLevel=”true”
logMessagesAtTransportLevel=”false”
maxMessagesToLog=”3000″
maxSizeOfMessageToLog=”2000″/>
</diagnostics>
</system.serviceModel>
NOTES:
logEntireMessage: By default, only the message header is logged but if we set it to true, entire message including message header as well as body will be logged.
logMalformedMessages: this option log messages those are rejected by WCF stack at any stage are known as malformed messages.
logMessagesAtServiceLevel: messages those are about to enter or leave user code.
logMessagesAtTransportLevel: messages those are about to encode or decode.
maxMessagesToLog: maximum quota for messages. Default value is 10000.
maxSizeOfMessageToLog: message size in bytes.
If you put all of the steps together, configuration file will appear like this:
<system.diagnostics>
<sources>
<source name=”System.ServiceModel.MessageLogging”>
<listeners>
<add name=”messagelistener”
type=”System.Diagnostics.XmlWriterTraceListener” initializeData=”d:logsmyMessages.svclog”></add>
</listeners>
</source>
</sources>
</system.diagnostics>
<system.serviceModel>
<diagnostics>
<messageLogging logEntireMessage=”true”
logMessagesAtServiceLevel=”false”
logMessagesAtTransportLevel=”false”
logMalformedMessages=”true”
maxMessagesToLog=”5000″
maxSizeOfMessageToLog=”2000″>
</messageLogging>
</diagnostics>
</system.serviceModel>
In this case, information will be buffered and not published to file automatically, So, we can set the autoflush property of the trace under sources as follows:
autoflush =”true” />
HostForLIFE.eu WCF Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
