
April 14, 2016 20:59 by
Peter
In this tutorial, I will show you how to create Web Services Using C#. This service will help you to communicate with the server.Windows Communication Foundation (WCF) is a framework for building service-oriented applications.
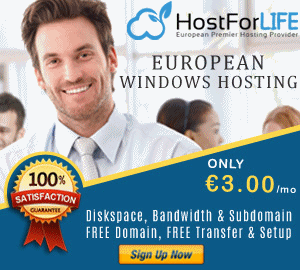
Using WCF, you can send data as asynchronous messages from one service endpoint to another. A service endpoint can be part of a continuously available service hosted by IIS, or it can be a service hosted in an application. An endpoint can be a client of a service that requests data from a service endpoint. The messages can be as simple as a single character or word sent as XML, or as complex as a stream of binary data.
Now, write the following code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Services;
using EntityLayer;
using BusinessLayer;
namespace WebApplication1
{
/// <summary>
/// Summary description for WebService1
/// </summary>
[WebService(Namespace = "http://hostforlife.eu")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
[System.ComponentModel.ToolboxItem(false)]
// To allow this Web Service to be called from script, using ASP.NET AJAX, uncomment the following line.
// [System.Web.Script.Services.ScriptService]
public class WebService1: System.Web.Services.WebService
{
[WebMethod]
public PurchaseEntity item(PurchaseEntity Vp)
{
PurchaseBusiness ObjectPurchaseOrder = new PurchaseBusiness();
return ObjectPurchaseOrder.item(Vp);
}
[WebMethod]
public PurchaseEntity Insert(PurchaseEntity obj1)
{
PurchaseBusiness ObjectPurchaseOrder = new PurchaseBusiness();
return ObjectPurchaseOrder.Insert(obj1);
}
[WebMethod]
public PurchaseEntity Delete(PurchaseEntity obj2)
{
PurchaseBusiness ObjectPurchaseOrder = new PurchaseBusiness();
return ObjectPurchaseOrder.Delete(obj2);
}
[WebMethod]
public PurchaseEntity Update(PurchaseEntity pe)
{
PurchaseBusiness ObjectPurchaseOrder = new PurchaseBusiness();
return ObjectPurchaseOrder.Update(pe);
}
}
}
HostForLIFE.eu WCF Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes.
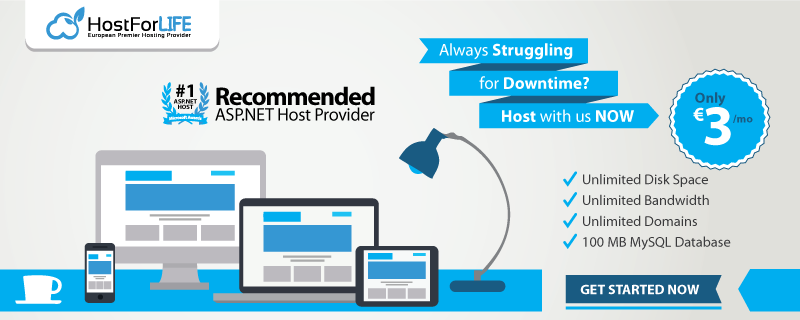