
February 27, 2019 11:25 by
Peter
The difference between TRUNCATE, DELETE, and DROP is one of the most common interview question. Here are some of the common differences between them.
TRUNCATE
TRUNCATE SQL query removes all rows from a table, without logging the individual row deletions.
The following example removes all data from the Customers table.
TRUNCATE TABLE Customers;
TRUNCATE is a DDL command
TRUNCATE is executed using a table lock and whole table is locked for remove all records.
We cannot use WHERE clause with TRUNCATE.
TRUNCATE removes all rows from a table.
Minimal logging in transaction log, so it is performance wise faster.
TRUNCATE TABLE removes the data by deallocating the data pages used to store the table data and records only the page deallocations in the transaction log.
Identify column is reset to its seed value if table contains any identity column.
To use Truncate on a table you need at least ALTER permission on the table.
Truncate uses the less transaction space than Delete statement.
Truncate cannot be used with indexed views.
TRUNCATE is faster than DELETE.
DELETE
To execute a DELETE queue, delete permissions are required on the target table. If you need to use a WHERE clause in a DELETE, select permissions are required as well.
The following query deletes all rows from the Customers table.
DELETE FROM Customers;
GO
The following SQL query deletes all rows from the Customers table where OrderID is greater than 1000.
DELETE FROM Customers WHERE OrderId > 1000;
GO
DELETE is a DML command.
DELETE is executed using a row lock, each row in the table is locked for deletion.
We can use where clause with DELETE to filter & delete specific records.
The DELETE command is used to remove rows from a table based on WHERE condition.
It maintain the log, so it slower than TRUNCATE.
The DELETE statement removes rows one at a time and records an entry in the transaction log for each deleted row.
Identity of column keep DELETE retain the identity.
To use Delete you need DELETE permission on the table.
Delete uses the more transaction space than Truncate statement.
Delete can be used with indexed views.
DROP
DROP table query removes one or more table definitions and all data, indexes, triggers, constraints, and permission specifications for those tables. DROP command requires ALTER permission on the schema to which the table belongs, CONTROL permission on the table, or membership in the db_ddladmin fixed database role.
The following SQL query drops the Customers table and its data and indexes from the current database.
DROP TABLE Customers ;
The DROP command removes a table from the database.
All the tables' rows, indexes and privileges will also be removed.
No DML triggers will be fired.
The operation cannot be rolled back.
DROP and TRUNCATE are DDL commands, whereas DELETE is a DML command.
DELETE operations can be rolled back (undone), while DROP and TRUNCATE operations cannot be rolled back
HostForLIFE.eu SQL Server 2016 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes.
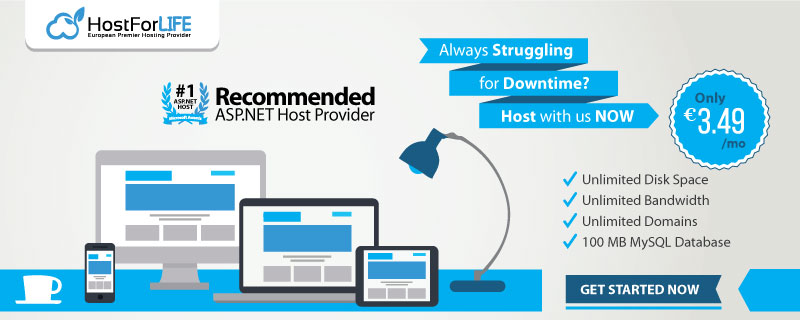

February 20, 2019 10:22 by
Peter
With the release of SQL Server 2017, a new TRIM() is also introduced which helps to remove the white space/characters from both sides of a string. Before 2017, this functionality was achieved by using the following SQL functions.
REPLACE - used o replace a character from a string
LTRIM - trim the white spaces from the left side of a string
RTRIM - trim the white spaces from the right side of a string
I can explain the functionality with two scenarios.
Let's assume, we have a string named ' ABC ' and we are going to eliminate the white spaces from both sides of the string.
In SQL, we usually use the LTRIM and RTRIM function like in the code below.
SELECT LTRIM( RTRIM(' ABC '))
Now, this can be done by using a single TRIM function.
SELECT TRIM(' ABC ')
Test results from SSMS can be seen below.
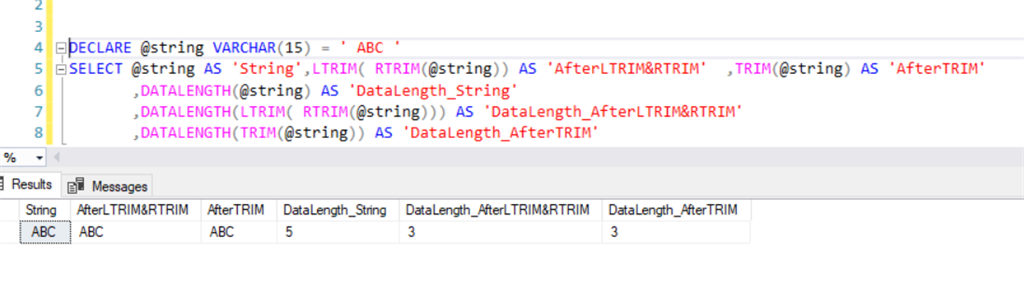
Assume we have a string named 'X ABC Y' and we need to extract 'ABC' from that. As usual, we will go with the REPLACE function as follows.
SELECT REPLACE(REPLACE('X ABC Y','X ',''),' Y','')
Here you go with the TRIM function.
SELECT TRIM('XY ' FROM 'X ABC Y')
Test results from SSMS are shown below.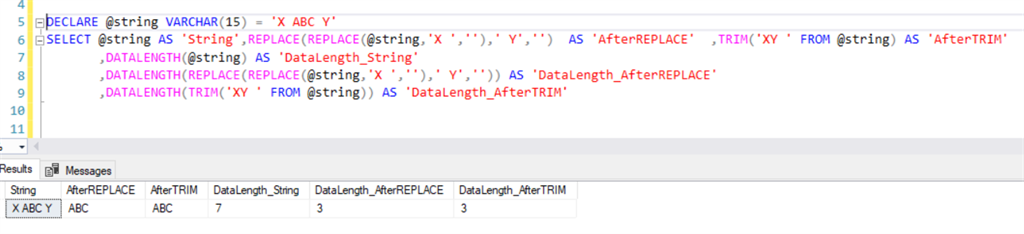
Note - It is necessary that you have to mention the trailing charter in the TRIM function, otherwise, this will not work as expected.
For example, if you try to remove the 'white space' only from the string 'X ABC Y', then TRIM will not help you. Similarly, if you don't mention the letter 'Y', TRIM will not remove the white space after the string, even though you already mentioned the 'X' and the 'white space' characters inside the TRIM function. See these scenarios in the below screenshot.
Test results from SSMS,
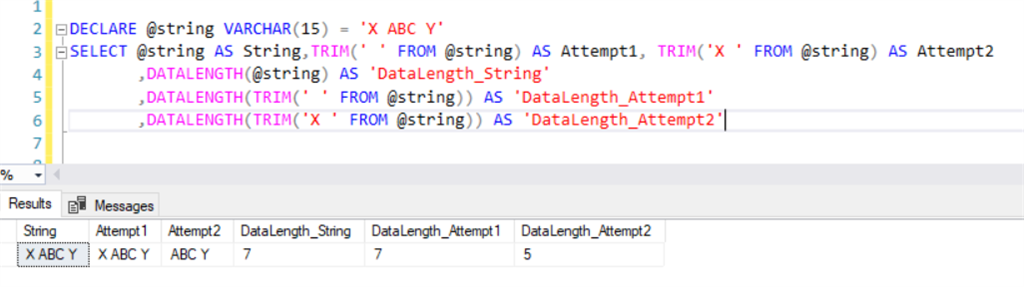
HostForLIFE.eu SQL Server 2016 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes.
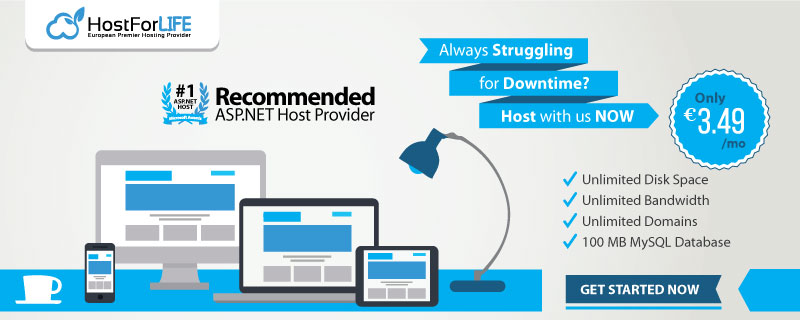

February 15, 2019 10:15 by
Peter
Most of the time, a developer requires encryption and decryption for encoding a message or information in such a way that only authorized person can retrieve that information.
In this article, I will discuss the implementation of AES encryption and decryption with Angular 7 while developing an application.
So, the first question that comes in our mind is "What is AES?".
AES stands for "Advanced Encryption Standard".
It is a tool that is used to encrypt and decrypt the simple text using AES encryption algorithm.
This algorithm was developed by two Belgian cryptographers, Joan Daemen and Vincent Rijmen.
AES was designed to be efficient in both, hardware and software, and supports a block length of 128 bits and key lengths of 128, 192, and 256 bits.
When to use AES Encryption
When you want to encrypt a confidential text into a decryptable format, for example - when you need to send some sensitive data in an e-mail.
The decryption of the encrypted text is possible only if you know the right password.
Now, try to implement the AES encryption and decryption in Angular 7. It's very easy to implement in Angular 7 with the help of crypto-js.
First, we create a new project with the following command.
ng new EncryptionDescryptionSample
After that, install crypto.js file, by the following command.
npm install crypto-js --save
For better UI, we also install bootstrap by the following command.
npm install bootstrap --save
After running the above command, check the package.json file if it is installed or not. Your file should look like this.
AES Encryption/Decryption with Angular 7
Now, go to "app.component.html" file and replace the existing code with the below code.
<h1 class="text-center">Encrypt-Decrypt with AES</h1>
<br>
<div>
<div class="row">
<div class="col-sm-6">
<button type="button" class="btn btn-primary btn-lg btn-block">
Encryption
</button>
<br>
<div class="form-group">
<label for="txtTextToConvert">Plain Text</label>
<input type="text" class="form-control" placeholder="Enter text you want to encrypt" [(ngModel)]="plainText">
</div>
<div class="form-group">
<label for="txtPassword">Password</label>
<input type="password" class="form-control" placeholder="Enter encryption password" [(ngModel)]="encPassword">
</div>
<textarea class="form-control" readonly rows="3">{{conversionEncryptOutput}}</textarea>
<br>
<button type="button" class="btn btn-success float-right" (click)="convertText('encrypt')">Encrypt</button>
</div>
<div class="col-sm-6">
<button type="button" class="btn btn-dark btn-lg btn-block">
Decryption
</button>
<br>
<div class="form-group">
<label for="txtTextToConvert">Encrypted Text</label>
<input type="text" class="form-control" placeholder="Enter text you want to decrypt" [(ngModel)]="encryptText">
</div>
<div class="form-group">
<label for="txtPassword">Password</label>
<input type="password" class="form-control" placeholder="Enter decryption password" [(ngModel)]="decPassword">
</div>
<textarea class="form-control" readonly rows="3">{{conversionDecryptOutput}}</textarea>
<br>
<button type="button" class="btn btn-success float-right" (click)="convertText('decrypt')">Decrypt</button>
</div>
</div>
</div>
Now, visit "app.component.ts" file and write the below code,
import { Component } from '@angular/core';
import * as CryptoJS from 'crypto-js';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'EncryptionDecryptionSample';
plainText:string;
encryptText: string;
encPassword: string;
decPassword:string;
conversionEncryptOutput: string;
conversionDecryptOutput:string;
constructor() {
}
//method is used to encrypt and decrypt the text
convertText(conversion:string) {
if (conversion=="encrypt") {
this.conversionEncryptOutput = CryptoJS.AES.encrypt(this.plainText.trim(), this.encPassword.trim()).toString();
}
else {
this.conversionDecryptOutput = CryptoJS.AES.decrypt(this.encryptText.trim(), this.decPassword.trim()).toString(CryptoJS.enc.Utf8);
}
}
}
In the above code, we used the Encrypt method of AES and passed our plain text with a password to encrypt the string. Similarly, we used the Decrypt method of AES and passed our encrypted text with a password to decrypt the string. Be sure that both times, your password is the same. For using both of these methods, you need to import "CryptoJS" from crypto-js.
import * as CryptoJS from 'crypto-js';
Now, let us run the project by using the following command.
ng serve --o
HostForLIFE.eu AngularJS Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes.


February 12, 2019 10:42 by
Peter
If you want to find a record in a table without having an index, the system will scan the whole table to get that record. Well, this is a very costly operation in terms of time. For example, if your table has 10 million records, it will scan all these records to find the value. To overcome this problem, indexes are used. Indexes use the B-TREE search algorithm to search a record in a table, which is way faster than the table scan.
Types of Index
Below are the frequently used types of Indexes.
- Clustered Index
A clustered index is an index that defines the physical order of records to be stored in a table. Therefore, the table can have only one clustered index.
- Non-Clustered Index
A non-clustered index is similar to an index in a book. The data is stored in one place and the index is stored in another place. Here, the index has pointers to the storage location of the data. Therefore, a table can have more than one Non-clustered index.
The syntax for creating an index.
CREATE INDEX <Index Name>
ON <Table Name> (<Column Name>);
/*Example of creating index on Lastname column of Persons table*/
CREATE INDEX idx_lastname
ON Persons (LastName);
Benefits of indexing foreign key columns
There are two primary benefits of using the Index on foreign key.
- Makes delete operation of parent table faster
When you delete a row from a parent table, the SQL Server checks if there are any rows which reference the row being deleted in the child table. To find the rows efficiently, an index on the foreign key column is extremely useful.
- Making joins faster
Using Index, SQL Server can more effectively find the rows to join to when the child and parent tables are joined.
Can we have multiple indexes on the same column?
- As you can have only one clustered index per table, you cannot have multiple clustered indexes on the same column; however, you can have multiple non-clustered indices on the same column.
HostForLIFE.eu SQL Server 2016 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes.
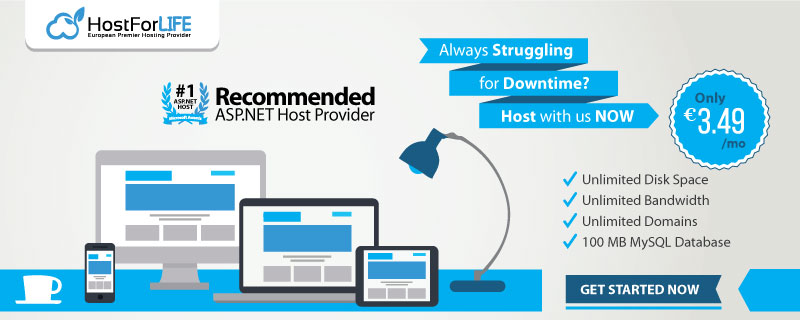