AngularJS Introduction
AngularJs is an open source Javascript framework to organise and assist web applications and single page applications. In 2012 we witnessed rise of Javascript MVC frameworks and libraries including Backbone.js , Ember.js and Angular.js.
AngularJS is created by Google to build single page applications which could be more architectured and maintainable. AngularJS is completely client-side and entirely JavaScript, so wherever Javascript runs AngularJS also runs. It is even less than 29kb making it highly minified and compressed. Angular is the next generation framework , in which every tool is designed such that it works with every other tool in an interconnected way.
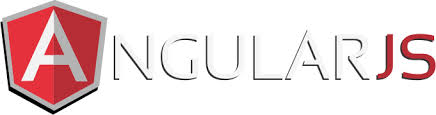
Interesting Point Using AngularJS
Angular has some compelling features for not just the developers but for designers as well.
Two Way Data Binding
It is the most crucial and useful feature of Angular. This feature is what all modern web apps are all about i.e Real Time. Two way binding permanently binds the view to the model and reduces refresh cycles, it also saves a considerable amount of code as previously 80% of code was dedicated to manipulating, traversing and listening to DOM . With data binding this code disappears and hence more concentration can be laid to application. Normally with change in model the DOM elements and attributes need to be manually manipulated to reflect the changes, it proves to be a complex process mainly when application grows in complexity and in size. But with two way data binding the synchronization between the DOM and the model is well taken care of.
HTML Template
AngularJS doesn’t rely on any rendering engine but uses browser parseable .html files for its partials. The HTML templates are parsed by the browser in the DOM. The DOM is now the input to the AngularJS compiler . Angular then traverses the DOM template for rendering called the Directives. The input here is bowser DOM and not the HTML string , this is the noticeable change between angular and all fellow frameworks.
Directives
Directives are stand alone reusable elements separated from the app . All DOM manipulations are performed by Directives. Directives are used to create custom HTML tags to serve as new custom widgets .With Directive you can create a new HTML tag or attribute and make it do anything you want . Directives are very unique, useful, powerful and reusable feature available only in angular. With Directives you can invent new HTML syntax that are specific to your application.
Dependency Injection
Dependency Injection is an angular feature that enables developers to easily build, develop, test and manage applications . With this feature you merely ask for for the dependencies instead of making manually , it will provide you an instance for any service asked provided you add the service as a parameter to get access to this service.
Testing
AngularJS is designed by keeping testability in mind such that angular applications can be tested easily as any Javascript code comes with a strong set of tests . Angular comes with a end to end test runner setup .
Why Use AngularJS?
AngularJS is a new Javascript framework by Google and is designed to make front end development easier . The popularity of single page applications and angular is flaming. Angular provides numerous concepts to to organize and manipulate the web applications.
Enabling a Parallel Workflow
It enables a parallel workflow between designers and developers. For a project both designing and hard coded developing can go side by side. For a project that is estimated to be completed in 4 months then by following the traditional sequential approach there would be dedicated 4 months of design followed by 4 months of coding making it 8 months altogether. But XAML allows to work in parallel by agreeing upon an interface for a screen. Developers can work on grabbing the data and writing all properties and tests around them while designers can animate and manipulate until they reach their final desired design . Those not familiar with XAML it is a declarative XML based language to instantiate object graphs and set values. The reason XML became so popular is because they translate well to angular.
Handling Dependencies
AngularJS easily handles dependency injections , angular lets you divide your app into modules that are initialized separately and having dependencies on each other. This enables you to test only the modules you want at once while also unfolding the ability to create end to end tests as well.
Dynamic loading is used by single page applications to deliver native app feel, but it involves a lot of dependencies on various modules and services, angular organises these and even manages the lifetime of an object for you.
Declarative UI
Having a declarative UI has many advantages associated with it. A structured UI is always easier to understand and manipulate. Without ,then by mere looking at the markup it can’t be figured what UI will actually do. So its not apparent whether any translations and validations are taking place by looking at some form tags.
But by declaring UI and by directly placing markup in HTML one can understand the extended markup angular provides. It makes it clear where and to what data is being bound to. With added tools like filters and directives the intent of UI is much clearer.
Development <-> Design Workflow
This works very well with angular, markup can be added without breaking an application as it depends on a particular structure or id to locate element and do task. Even rearrangement of code is much easier as the corresponding code that binds with it also moves along.
Flexibility with Filters
Filters are standalone functions and filter the data before reaching the view, it can involve formatting decimal places or reversing an array or simply implementing pagination. Filters are separate from app just like directives and are so resourceful that creation of a sorted HTML table is possible with filters without writing any Javascript.
These fundamental features and principles will let you create a performance driven, maintainable , extensible and efficient front end codebase . AngularJS provides a rich experience to the end user, it is a robust and well maintained Javascript framework suitable for any professional web development.