Today, i'm gonna show you how to get started with OWIN and Katana Project. Open Web Interface for .NET (OWIN) is a specification for the interaction between web servers and web applications. It has its roots in the open source community. By decoupling the web server from the application, OWIN makes it easier to create middleware for .NET web development. Also, OWIN makes it easier to port web applications to other hosts, for example, self-hosting in a Windows service or other process. OWIN is a community-owned specification, not an implementation. The implementation of OWIN is called as Katana Project. The Katana project is a set of open-source OWIN components developed by Microsoft.
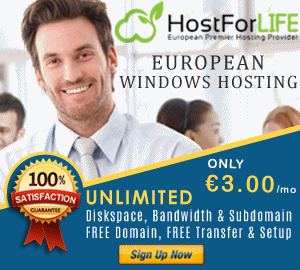
Katana is a webframework which is really lightweight and much more modular then ASP.NET. ASP.NET applications always include System.Web, which contains all functionality like caching, authorization, etc. Katana is way more modular. It let’s you build a application where you only add the references you really need. Katana lets you compose a modern Web application from a wide range of different Web technologies and then host that application wherever you wish, exposed under a single HTTP endpoint. This provides several benefits:
- Deployment is easy because it involves only a single application rather than one application per capability.
- You can add additional capabilities, such as authentication, which can apply to all of the downstream components in the pipeline.
- Different components, whether Microsoft or third-party, can operate on the same request state via the environment dictionary.
So, Katana is a flexible set of components for building and hosting OWIN-based web applications. And this post is just a quick start to give you an impression of the power that Katana gives you.
The code below shows how easy is to get started with Katana. Create a new console application and install the nuget package: Microsoft.Owin.SelfHost. And If you now paste the code, you will already have a working website at localhost:8080.
class Program
{
static void Main(string[] args)
{
var url = "http://localhost:8080";
using (WebApp.Start<Startup>(url))
{
Console.ReadLine();
}
}
}
public class Startup
{
public void Configuration(IAppBuilder app)
{
app.Run(x =>
{
x.Response.ContentType = "text/plain";
return x.Response.WriteAsync("Hello world");
});
}
}
We define a url and a startup class. The Startup class needs a configuration method in which we can set the run method with a Func on your IAppBuilder. This appbuilder is the “configuration” of your web application and the run method adds a piece of middleware to your OWIN pipeline which doesn’t invoke a next piece of middleware. Look at it at as the endpoint of all the web requests for this small web application.
The appbuilder also allows you to add more middleware to your OWIN pipeline. If we for instance want to have some middleware that handles cookies we can add it with a few lines of code.
public class CookieMonster : OwinMiddleware
{
public CookieMonster(OwinMiddleware next) : base(next)
{
}
public async override Task Invoke(IOwinContext context)
{
context.Response.Cookies.Append("testcookie", "hello world");
await Next.Invoke(context);
}
}
And now, we need to inherit from OwinMiddleware, add a constructor and a async Invoke method. In the invoke method we add our cookie (or whatever logic you want) and make it invoke the next middleware down the pipeline. To plug this middleware into our application, we only need our appbuilder to use it. Just add the following line in your Startup class above the app.Run line and your done!!
app.Use<CookieMonster>();
Now, you have a working Katana Web Application that adds an annoying cookie. Easy right? This was a really simplified example and there is a lot more to Katana.
For the best OWIN and Katana.NET Hosting, HostForLIFE.eu is the right answer!
HostForLIFE.eu OWIN and Katana.NET Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes. We have customers from around the globe, spread across every continent. We serve the hosting needs of the business and professional, government and nonprofit, entertainment and personal use market segments.
