
May 12, 2016 00:08 by
Peter
In this article I am going to explain how to save the youtube into database and display it in Gridview using Linq. I have got a requirement to save the youtube video URL into database and display them where user can play the videos. I have created a table Tb_videos which store the information video tile, description and URL as you can see on the following :
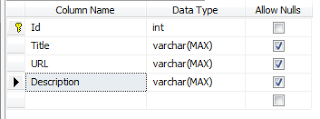
HTML Markup:
<table>
<tr>
<td>Title :</td>
<td>
<asp:TextBox ID="txttitle" runat="server" Width="450px"></asp:TextBox></td>
</tr>
<tr>
<td></td>
<td></td>
</tr>
<tr>
<td>Description :</td>
<td><asp:TextBox ID="txtdescription" runat="server" TextMode="MultiLine" Width="450px"></asp:TextBox></td>
</tr>
<tr>
<td></td>
<td></td>
</tr>
<tr>
<td>Video URL :</td>
<td><asp:TextBox ID="txturl" runat="server" Width="450px"></asp:TextBox></td>
</tr>
<tr>
<td></td>
<td></td>
</tr>
<tr>
<td></td>
<td>
<asp:Button ID="btnsubmit" runat="server" Text="Submit" /></td>
</tr>
</table>
<br />
<asp:GridView ID="grdvideo" runat="server" AutoGenerateColumns="False"
BackColor="White" BorderColor="#DEDFDE" BorderStyle="None" BorderWidth="1px"
CellPadding="4" ForeColor="Black" GridLines="Vertical">
<AlternatingRowStyle BackColor="White" />
<Columns>
<asp:BoundField DataField="Title" HeaderText="Title" />
<asp:BoundField DataField="Description" HeaderText="Description"/>
<asp:TemplateField HeaderText="Videos">
<ItemTemplate>
<object width="480" height="385">
<embed src='<%#Eval("url") %>' type="application/x-shockwave-flash" allowscriptaccess="always" allowfullscreen="true" width="480" height="385">
</embed>
</object>
</ItemTemplate>
</asp:TemplateField>
</Columns>
<FooterStyle BackColor="#CCCC99" />
<HeaderStyle BackColor="#6B696B" Font-Bold="True" ForeColor="White" />
<PagerStyle BackColor="#F7F7DE" ForeColor="Black" HorizontalAlign="Right" />
<RowStyle BackColor="#F7F7DE" />
<SelectedRowStyle BackColor="#CE5D5A" Font-Bold="True" ForeColor="White" />
<SortedAscendingCellStyle BackColor="#FBFBF2" />
<SortedAscendingHeaderStyle BackColor="#848384" />
<SortedDescendingCellStyle BackColor="#EAEAD3" />
<SortedDescendingHeaderStyle BackColor="#575357" />
</asp:GridView>
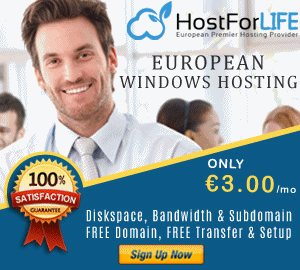
Import the namespace
C#:
using System.Text.RegularExpressions;
VB:
Imports System.Text.RegularExpressions
Create the object of DBML:
C#:
BlogDataContext db = new BlogDataContext();
VB:
Private db As New BlogDataContext()
Get the Youtube video id
Write a function to get the youtube video id from youtube video URL .
C# Code:
private string GetYouTubeVideoID(string youTubeVideoUrl)
{
var regexMatch = Regex.Match(youTubeVideoUrl, "^[^v]+v=(.{11}).*",
RegexOptions.IgnoreCase);
if (regexMatch.Success)
{
return "http://www.youtube.com/v/" + regexMatch.Groups[1].Value +"&hl=en&fs=1";
}
return youTubeVideoUrl;
}
VB Code:
Private Function GetYouTubeVideoID(ByVal youTubeVideoUrl As String) As String
Dim regexMatch = Regex.Match(youTubeVideoUrl, "^[^v]+v=(.{11}).*", RegexOptions.IgnoreCase)
If regexMatch.Success Then
Return "http://www.youtube.com/v/" + regexMatch.Groups(1).Value + "&hl=en&fs=1"
End If
Return youTubeVideoUrl
End Function
Save the record to database
On button click write the below given code to insert the record into database table.
C# Code:
protected void btnsubmit_Click(object sender, EventArgs e)
{
try
{
string ytFormattedVideoUrl = GetYouTubeVideoID(txturl.Text);
Tb_Video tb = new Tb_Video();
tb.Title = txttitle.Text;
tb.Description = txtdescription.Text;
tb.URL = ytFormattedVideoUrl;
db.Tb_Videos.InsertOnSubmit(tb);
db.SubmitChanges();
Response.Write("<script>alert('Record Inserted Successfully');</script>");
BindGrid();
Clear();
}
catch (Exception ex)
{ }
}
public void Clear()
{
txturl.Text = string.Empty;
txtdescription.Text = string.Empty;
txttitle.Text = string.Empty;
}
VB Code:
Protected Sub btnsubmit_Click(ByVal sender As Object, ByVal e As System.EventArgs) Handles btnsubmit.Click
Try
Dim ytFormattedVideoUrl As String = GetYouTubeVideoID(txturl.Text)
Dim tb As New Tb_Video()
tb.Title = txttitle.Text
tb.Description = txtdescription.Text
tb.URL = ytFormattedVideoUrl
db.Tb_Videos.InsertOnSubmit(tb)
db.SubmitChanges()
Response.Write("<script>alert('Record Inserted Successfully');</script>")
BindGrid()
Clear()
Catch ex As Exception
End Try
End Sub
Public Sub Clear()
txturl.Text = String.Empty
txtdescription.Text = String.Empty
txttitle.Text = String.Empty
End Sub
Fetch the record from database and display
Create a method to get the record from database table and display in Gridview data control.
C# Code:
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
BindGrid();
}
}
public void BindGrid()
{
try
{
var bind = from v in db.Tb_Videos
select v;
grdvideo.DataSource = bind;
grdvideo.DataBind();
}
catch (Exception ex)
{
}
}
VB Code:
Protected Sub Page_Load(ByVal sender As Object, ByVal e As EventArgs) Handles Me.Load
If Not IsPostBack Then
BindGrid()
End If
End Sub
Public Sub BindGrid()
Try
Dim bind = From v In db.Tb_Videos
grdvideo.DataSource = bind
grdvideo.DataBind()
Catch ex As Exception
End Try
End Sub
Build the project and run. To test the application inserts a record into database.
HostForLIFE.eu SQL Server 2014 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes.
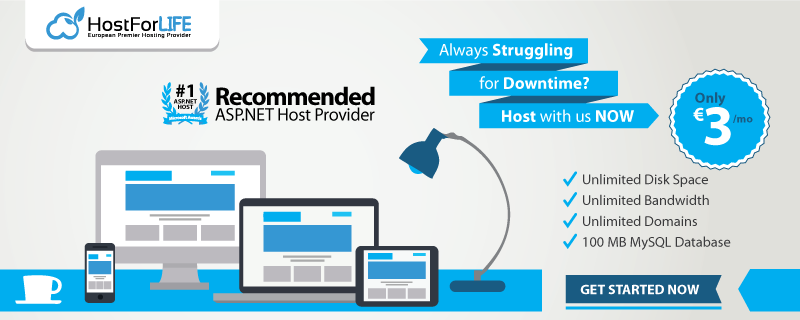