One of the most popular relational database management systems (RDBMS), SQL Server, has several strong features. The SELECT statement is one of the most important and commonly used commands among them. It is the foundation of the majority of SQL operations since it enables users to get data from a database. This article will examine SQL Server's SELECT capability, including its fundamental syntax, more complex applications, and some best practices for maximizing its use.
We demonstrate the results of these claims using a sample Employee table to help put this idea into concrete form.
SELECT Statement
To fetch all records from the Employees table.
SELECT * FROM Employees;
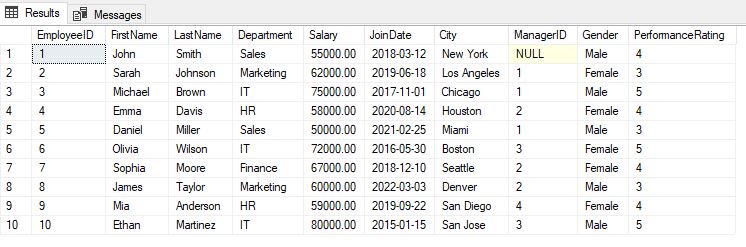
In this query
- SELECT * tells SQL Server to return all columns.
- FROM Employees specifies the table from which to retrieve the data.
While using * to select all columns can be convenient, it is generally better practice to specify the exact columns you need to retrieve. This reduces unnecessary data retrieval and improves performance, especially when dealing with large tables. At its core, the SELECT statement allows you to query data from one or more tables. The basic syntax for a SELECT query looks like this.
SELECT FirstName, LastName FROM Employees;
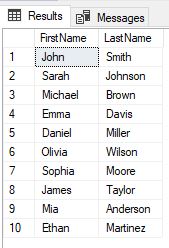
Filtering Data with the WHERE Clause
The real power of SELECT comes when you start using the WHERE clause to filter the data returned. This allows you to specify conditions that must be met for rows to be included in the result set.
SELECT FirstName, LastName FROM Employees
WHERE Department = 'Sales';
The query fetches only the employees working in the "Sales" department.
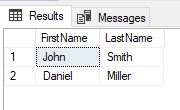
You can also use operators like =, >, <, BETWEEN, IN, LIKE, and IS NULL to create complex conditions. For example.
SELECT FirstName, LastName, Salary FROM Employees
WHERE Salary > 50000 AND Department IN ('HR', 'Sales');
This retrieves the employees in the HR or Sales departments with a salary greater than $50,000.
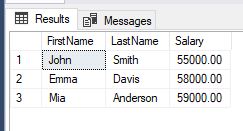
Sorting Data with ORDER BY
To arrange the result set in a specific order, you can use the ORDER BY clause. By default, it sorts in ascending order (A to Z, lowest to highest), but you can also use DESC to sort in descending order.
SELECT FirstName, LastName, Salary FROM Employees
ORDER BY Salary DESC;
This query returns the employees sorted by salary, from the highest to the lowest.
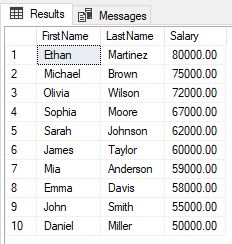
Aggregating Data with GROUP BY
In many cases, you'll want to group rows together based on certain columns and then perform aggregate functions like COUNT, SUM, AVG, MIN, or MAX on those groups. The GROUP BY clause allows you to do this.
SELECT Department, COUNT(*) AS EmployeeCount
FROM Employees
GROUP BY Department;
This query returns the number of employees in each department. The COUNT(*) function counts the number of rows in each group formed by the Department column.
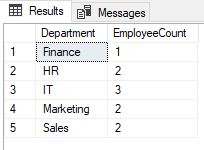
Using DISTINCT to Remove Duplicates
If you want to eliminate duplicate rows from your result set, you can use the DISTINCT keyword. This is particularly useful when querying data that might contain repeated values.
SELECT DISTINCT Department FROM Employees;
This query returns only the unique departments from the Employees table.
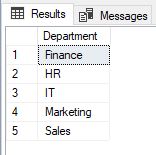
Limiting the Number of Results with TOP
When working with large datasets, it’s often useful to limit the number of rows returned by a query. SQL Server allows you to do this using the TOP keyword.
SELECT TOP 5 FirstName, LastName FROM Employees;
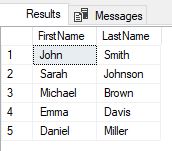
This query returns only the first 5 rows from the Employees table. You can also use PERCENT to return a percentage of the rows.
SELECT TOP 10 PERCENT FirstName, LastName FROM Employees;
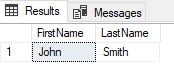
Subqueries and Nested Queries
SQL Server allows you to nest queries within other queries. A subquery is a query that is embedded inside a larger query. Subqueries can be used in the SELECT, FROM, and WHERE clauses.
SELECT FirstName, LastName, Salary
FROM Employees
WHERE Salary > (SELECT AVG(Salary) FROM Employees);
This query returns the names and salaries of employees who earn more than the average salary.
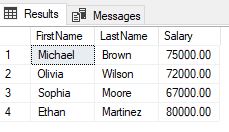
Best Practices for Using SELECT in SQL Server
- Be Specific with Columns: Avoid using SELECT * in production code, as it can lead to unnecessary data being returned and can negatively impact performance.
- Use Indexes Efficiently: Proper indexing on tables can significantly speed up SELECT queries, especially those with WHERE conditions or JOIN operations.
- Avoid N+1 Query Problem: When using JOIN or subqueries, ensure your queries are optimized to prevent fetching data multiple times unnecessarily.
- Limit Data Retrieval: When testing queries or working with large datasets, always limit the number of rows returned using TOP, especially if you don’t need the entire dataset.
Conclusion
The SELECT statement is a versatile and powerful feature in SQL Server that allows developers to retrieve and manipulate data effectively. By mastering its basic and advanced features, you can write efficient queries that meet your application’s needs. Whether you're filtering data, aggregating results, or joining multiple tables, understanding how to use SELECT to its full potential is crucial for any SQL Server user. By following best practices and leveraging the various clauses and functions available, you can optimize the performance of your queries and ensure that your database operations are both effective and efficient.
HostForLIFE.eu SQL Server 2022 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes.
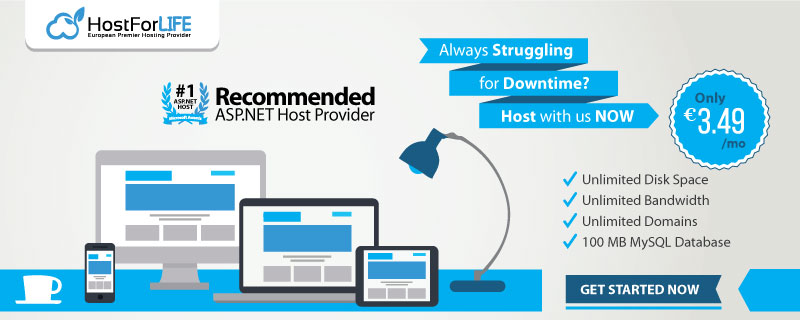