To continue the last tutorial about how to insert data page in ASP.NET Web Pages 3.1, today I'm gonna tell you how to read the data page that we have inserted with example code.
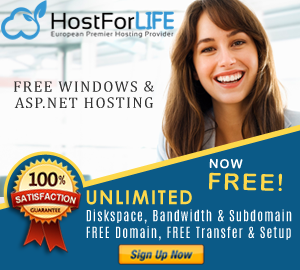
These are the steps to read the data page:
Step 1
The first thing to do is create a variable db which opens the database. Then, you will have another variable called GameName which is set to get the RouteValue from the URL (we will use routing for clean URLS). Beneath that is an If Statement which checks if GameName is null, if it is, the user is redirected back to the home page.
In the Read.cshtml file insert following code:
@{
var db = Database.Open("StarterSite");
var GameName = Context.GetRouteValue("GameName");
if (GameName == null){Response.Redirect("~/");}
var SQLREAD = "SELECT * From Games Where GameName=@0";
var data = db.QuerySingle(SQLREAD, GameName);
var Id = data.Id;
var Text = data.Text;
Page.Title = data.mTitle;
Page.mDescription = data.mDescription;
Step 2
Next you have a SQL command which will get the data from the database, the where clause is used to filter out results. The db.QuerySingle retrieves one result from the database, and we provide two arguments, the command and parameter, which is the GameName variable. The GameName variable stores the current page name. For example, our route has the following pattern:
RouteTable.Routes.MapWebPageRoute("games/{GameName}", "~/Read.cshtml");
When you go to an address like games/infamous the GameName variable has the value infamous, so the SQL command can be translated to:
var SQLREAD = "SELECT * From Games Where GameName=’infamous’";
Step 3
The data variable is now set to retrieve data from the database and only for the current page, since the where clause has filtered out the rows. Usually you only want to retrieve one row and place the data where you would like it to go. This is easily done when you type data after the dot (period): you specify the column name and the data for that row will be displayed. You can create the ID variable because it's a need to output the result a few times, as seen below.
<h2>@data.GameName</h2>
@Html.Raw(Text)
<span>@data.ReleaseDate - <a href="/update.cshtml?id=@Id">Edit Page</a> | <a href="/delete.cshtml?id=@Id&[email protected]">Delete Page</a></span>
The Html.Raw method will render the HTML properly, and this is another built-in ASP.NET security feature. If you do not use Html.Raw, you will get the HTML source printing out. The ?id=@id is a parameter and is used to pass data to the other pages. For example, when the user goes to the update page it will request the value of ID and will load that row from the database. So if ID= 1 it will load row 1. The same applies for delete page, although instead of updating the page it will be deleted. The returnurl as seen above is used to redirect the user back if the user rejects the confirmation to delete the page.
Here is the ouput example:
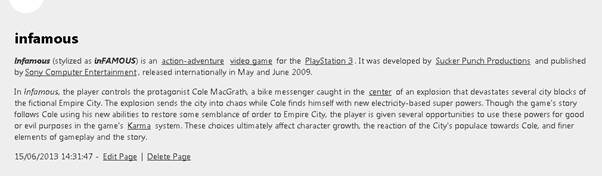
Step 4
Now we will add one more read data page. In the Default.cshtml page replace the code with this below:
@{
var db = Database.Open("StarterSite");
var SQLREAD = "SELECT * From Games";
var data = db.Query(SQLREAD);
}
<h2>Recent Games</h2>
<ul>
@foreach (var game in data)
{
<li><a href="/games/@game.GameName">@game.GameName</a></li>
}
</ul>
This time we used the db.Query method. This gets all the rows from the table. Then we displayed all the results in an unordered list, which will allow us to view the games list from the default page instead of having to type it in the address bar.
Here is the ouput example:
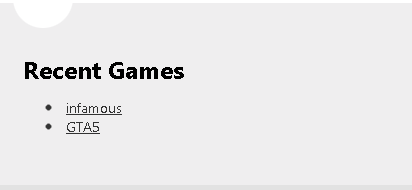
HostForLIFE.eu ASP.NET WebPages 3.1 with Free ASP.NET Hosting
Try our ASP.NET WebPages 3.1 with Free ASP.NET Hosting today and your account will be setup soon! You can also take advantage of our Windows & ASP.NET Hosting support with Unlimited Domain, Unlimited Bandwidth, Unlimited Disk Space, etc. You will not be charged a cent for trying our service for the next 3 days. Once your trial period is complete, you decide whether you'd like to continue.
