In this post, I will tell you how to insert data page with example code in ASP.NET Webpages 3.1.
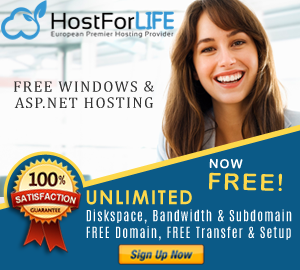
Follow these steps to insert data page:
Step 1
Click Databases. then click on Home in the ribbon menu, and Click on New Query. Let's copy this following code:
CREATE TABLE Games
(
Id INT NOT NULL PRIMARY KEY IDENTITY,
GameName NVARCHAR(200) NOT NULL,
ReleaseDate DateTime NOT NULL,
mTitle NVARCHAR(200) NOT NULL,
mDescription NVARCHAR(200) NOT NULL,
Text nText NOT NULL
);
Step 2
Click Execute.
Now, you have created a new table and a few columns so we can perform our CRUD operations. In the Create page copy the following:
@{
var GameName = "";
DateTime ReleaseDate = DateTime.UtcNow;
var mTitle = "";
var mDescription = "";
var Text ="";
Then you have created 5 variables which you will use to collect the user-entered data and then insert the data into a database. The second variable is a DateTime data type. The second column in the database is for DateTime. The user will not have to specify the ReleaseDate, as it has been set as the current UTC date and time.
if (IsPost)
{
GameName = Request["GameName"];
mTitle = Request["mTitle"];
mDescription = Request["mDescription"];
Text = Request.Unvalidated("Text");
Validation.RequireFields("GameName", "mTitle", "mDescription", "Text");
var SQLINSERT = "INSERT INTO Games (GameName, ReleaseDate, mTitle, mDescription, Text) VALUES (@0, @1, @2, @3, @4)";
When the user submits the data we request the values from the HTML elements. The Request.Unvalidated allows the user to type anything they like. By default, ASP.NET will not allow you to type HTML and JavaScript; this is a security measure to avoid XSS attacks.
The validation ensures that no field is left blank. You have to separate each field with a comma. Now, SQLINSERT is our database command. You can specify the column names and then the values which will be inserted. When we use @0, @1 we pass the data as parameters; this is the safest way to do this as it avoids XSS attacks. Always pass the data like this:
if (Validation.IsValid())
{
try
{
var db = Database.Open("StarterSite");
db.Execute(SQLINSERT, GameName, ReleaseDate, mTitle, mDescription, Text);
Response.Write("Data Saved!");
}catch (Exception ex)
{
Response.Write(ex.Message);
}
}
}
}
Lastly, we ensure that the data entered is valid, and then use a try catch block to execute the database command. The db.Execute method takes two arguments (string command, param object[] args); the second argument is basically the parameters. When you specify the parameters in the Execute method you need to make sure that it is in the right order, otherwise you will get errors. For example, try entering:
db.Execute(SQLINSERT, GameName, mTitle, ReleaseDate, mDescription, Text);
This will flag an error because the second column expects a data type of DateTime. It is good practise to keep the order of the columns as they appear in the table.
Full HTML Script
<form method="post">
<fieldset>
<legend>Insert Data</legend>
@Html.ValidationSummary(true)
<div>
<label>Game Name</label>
<input type="text" value="@GameName" name="GameName"/>
@Html.ValidationMessage("GameName")
</div>
<div>
<label>Meta Title</label>
<input type="text" value="@mTitle" name="mTitle"/>
@Html.ValidationMessage("mTitle")
</div>
<div>
<label>Meta Description</label>
<input type="text" value="@mDescription" name="mDescription"/>
@Html.ValidationMessage("mDescription")
</div>
<div>
<label>Text</label>
<textarea class="ckeditor" name="Text">@Text</textarea>
@Html.ValidationMessage("Text")
</div>
<input type="submit"/>
</fieldset>
</form>
<script src="/ckeditor/ckeditor.js"></script>
One thing that should be explained is the <script> tag. We will use CKEditor for our <textarea>, as this allows us to add HTML styles.
Finaaly, you can run the page and add a new entry, and remember the title for the GameName. Make sure the GameName does not have any spaces or characters; stick with numbers, letters and dashes only.
HostForLIFE.eu ASP.NET WebPages 3.1 with Free ASP.NET Hosting
Try our ASP.NET WebPages 3.1 with Free ASP.NET Hosting today and your account will be setup soon! You can also take advantage of our Windows & ASP.NET Hosting support with Unlimited Domain, Unlimited Bandwidth, Unlimited Disk Space, etc. You will not be charged a cent for trying our service for the next 3 days. Once your trial period is complete, you decide whether you'd like to continue.
