
March 24, 2025 08:29 by
Peter
Depending on the input values, scalar functions in SQL return a single value. Instead of working with sets of rows, these functions work with individual values.
Common Scalar Functions
LEN(): Returns the length of a string.
UPPER(): Converts a string to uppercase.
LOWER(): Converts a string to lowercase.
ROUND(): Rounds a number to a specified decimal place.
GETDATE(): Returns the current date and time.
Example Usage of Scalar Functions
1. Using LEN() Function
SELECT LEN('Hello World') AS StringLength;
2. Using UPPER() and LOWER() Functions
SELECT UPPER('hello') AS UpperCase, LOWER('WORLD') AS LowerCase;
Output
UpperCase | LowerCase |
HELLO |
world |
3. Using ROUND() Function
SELECT ROUND(123.456, 2) AS RoundedValue
4. Using GETDATE() Function
SELECT GETDATE() AS CurrentDateTime;
5. Using ABS() Function
SELECT ABS(-25) AS AbsoluteValue;
6. Using SQRT() Function
SELECT SQRT(49) AS SquareRoot;
7. Using SUBSTRING() Function
SELECT SUBSTRING('SQL Functions', 5, 9) AS SubstringResult;
8. Using REPLACE() Function
SELECT REPLACE('Hello SQL', 'SQL', 'World') AS ReplacedString;
Advanced Use of Scalar Functions
1. Combining Scalar Functions
SELECT UPPER(LEFT('advanced scalar functions', 8)) AS Result;
2. Using Scalar Functions in Computations
SELECT ROUND(AVG(Salary), 2) AS AverageSalary FROM Employees;
3. Formatting Dates Using Scalar Functions
SELECT FORMAT(GETDATE(), 'yyyy-MM-dd') AS FormattedDate;
4. Custom Scalar Function Example
CREATE FUNCTION dbo.Getfullname(@FirstName NVARCHAR(50),
@LastName NVARCHAR(50))
returns NVARCHAR(100)
AS
BEGIN
RETURN ( @FirstName + ' ' + @LastName )
END;
Usage
SELECT dbo.GetFullName('John', 'Doe') AS FullName;
Advantages of Scalar Functions
- Helps in data formatting and transformation.
- Improves code readability and maintainability.
- Enhances query flexibility with built-in SQL functions.
Scalar functions are essential for manipulating individual values in SQL queries.
HostForLIFE.eu SQL Server 2022 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes.
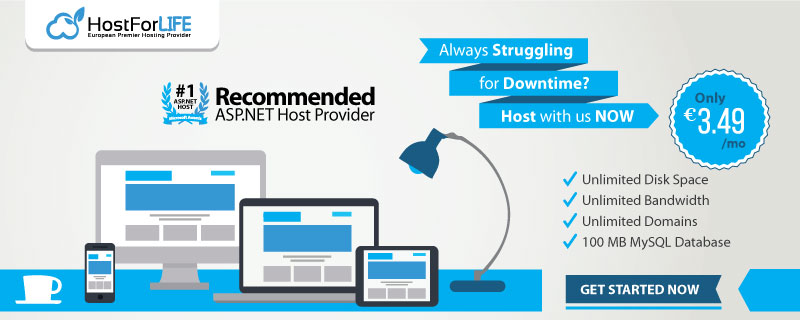