
December 11, 2023 07:15 by
Peter
Overview
Microsoft SQL Server is a well-known and potent relational database solution in the field of database management. During their exploration of the complex world of SQL, developers and database administrators often find themselves returning to the same questions in both interviews and practical situations.
We'll explore the most commonly asked SQL questions for MS SQL Server in this blog, offering tips and code samples to help novices and experts alike.
Retrieving Data. The SELECT Statement
The foundation of any database interaction lies in retrieving data. The SELECT statement is your go-to tool for this task.
-- Retrieve all columns from a table
SELECT * FROM TableName;
-- Retrieve specific columns
SELECT Column1, Column2 FROM TableName;
-- Filter data with WHERE clause
SELECT * FROM TableName WHERE Condition;
Filtering and Sorting Data
Refining data based on conditions and sorting results is a common requirement.
-- Filtering with WHERE
SELECT * FROM TableName WHERE Column = 'Value';
-- Sorting with ORDER BY
SELECT * FROM TableName ORDER BY Column ASC/DESC;
Aggregating Data. GROUP BY and Aggregate Functions
Aggregating data provides valuable insights into summaries and statistics.
-- Grouping data with GROUP BY
SELECT Column, COUNT(*)
FROM TableName
GROUP BY Column;
-- Using Aggregate Functions
SELECT AVG(Column), SUM(Column), MAX(Column)
FROM TableName
WHERE Condition;
Joining Tables. INNER JOIN, LEFT JOIN
When data resides in multiple tables, joining becomes essential.
-- Inner Join
SELECT *
FROM Table1
INNER JOIN Table2 ON Table1.ID = Table2.ID;
-- Left Join
SELECT *
FROM Table1
LEFT JOIN Table2 ON Table1.ID = Table2.ID;
Subqueries. Nesting Queries for Precision
Subqueries enable embedding one query within another, offering precision and flexibility.
-- Using Subqueries
SELECT *
FROM TableName
WHERE Column IN (SELECT Column FROM AnotherTable WHERE Condition);
Modifying Data. UPDATE and DELETE Statements
Ensuring data accuracy involves updating and deleting records.
-- Update Statement
UPDATE TableName SET Column = 'NewValue' WHERE Condition;
-- Delete Statement
DELETE FROM TableName WHERE Condition;
Transaction Control. BEGIN, COMMIT, ROLLBACK
Transactions maintain data integrity by grouping operations.
-- Begin Transaction
BEGIN TRANSACTION;
-- Commit Transaction
COMMIT;
-- Rollback Transaction
ROLLBACK;
Working with Dates. Date Functions
Manipulating dates is a common task, and SQL Server provides robust date functions.
-- Get Current Date
SELECT GETDATE();
-- Extract Part of a Date
SELECT YEAR(DateColumn), MONTH(DateColumn), DAY(DateColumn);
Common Table Expressions (CTEs). Enhancing Readability
CTEs simplify complex queries and enhance code readability.
-- Using CTE
WITH MyCTE AS (
SELECT Column FROM TableName WHERE Condition
)
SELECT * FROM MyCTE;
Window Functions. Analytical Insights
Window functions offer advanced analytical capabilities.
-- Using Window Function
SELECT Column, ROW_NUMBER() OVER (ORDER BY Column) AS RowNum
FROM TableName;
These SQL queries encapsulate the essence of database interactions and form the backbone of database-related interviews and projects. Mastering these queries empowers professionals to navigate diverse scenarios efficiently.
Conclusion
The world of SQL Server queries is vast, and continuous learning is key.
As you embark on your SQL journey, these fundamental queries will serve as the stepping stones to deeper insights and mastery. Happy querying!
HostForLIFE.eu SQL Server 2022 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes.
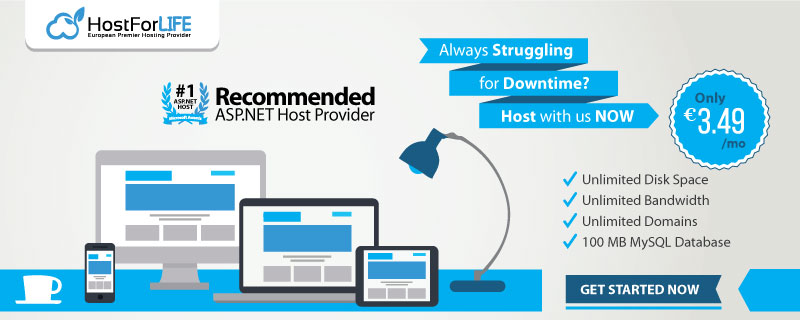