HTTP server was ok for us to try and do numerous operations that we've performed within the articles lately. During this specific article however, we are going to be looking at a way to create HTTPS server instead with Node.js.
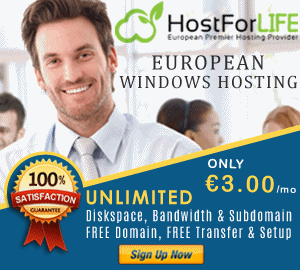
We use HTTPS after we would like secure sessions. A secure session implies that the online browser can encrypt everything you are doing with a digitally signed certificate. Therefore clearly before you are doing HTTPS, you would like to make certificates. Let's therefore spend a while to check a way to develop certificates for SSL.
Make a SSL Certificates
In order to make a certificate, you need to download a small tool OpenSSL first from this link https://code.google.com/p/openssl-for-windows/downloads/list
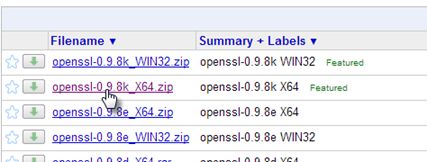
Download the version as per your system's specification, it's essentially a zip file. Once downloaded, extract the content to a such location onto your disc drive. Now open the location of the extracted content and copy the file openssl.cnf to the bin folder.
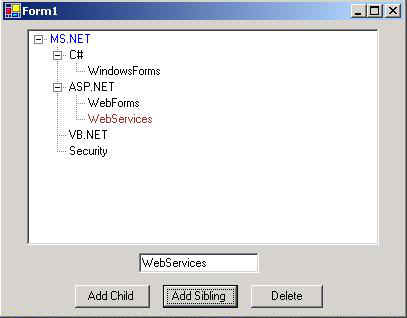
That is the configuration file for the Openssl. Next, open CMD, change the directory to this folder and execute the following code.
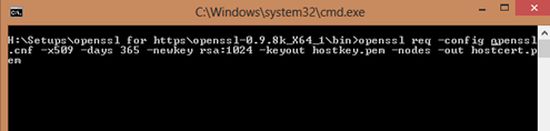
openssl req -config openssl.cnf -x509 -days 365 -newkey rsa:1024 -keyout hostkey.pem -nodes -out hostcert.pem
The program can then a few you for a few peices of data for creating the certificate. in the end of everything, you may have 2 files hostcert.pem and hostkey.pem, that is our certificate and key file respectively, that we are going to be using in our HTTPS server.
Creating HTTPS server
Just like we have a tendency to do for HTTP, an import to node's HTTP module, for HTTPS we import the HTTPS module. Also, since we'd like to pass within the certificate and key file, we also need to import the filestream (fs) module to enable Node to read the files. the following is that the code to make the HTTPS server.
var https = require('https');
var fs = require('fs');
var options = {
key: fs.readFileSync('hostkey.pem'),
cert: fs.readFileSync('hostcert.pem')
};
https.createServer(options, function (req, res) {
res.writeHead(200);
res.end("hello world\n");
}).listen(8000);
Of course you wish to copy the files into the same location because the node program. now if you explore the code above, you will find that it's very similar to what we discussed before, like reading files, making a http server so on. The change however is that the https.createServer() method that takes another parameter, that is that the certificate and key for the SSL.
Now if you run the code and see it in a browser, this can be how it looks:
Select proceed anyway option as of now, as our browser does not understand our amazing certificate.