
June 18, 2015 07:18 by
Peter
This article will explains the way to Save pictures In Sqlserver database In Asp.Net using File upload control. I am upload the pictures using FileUpload control and saving or storing them in SQL Server database in ASP.NET with C# and VB.NET.
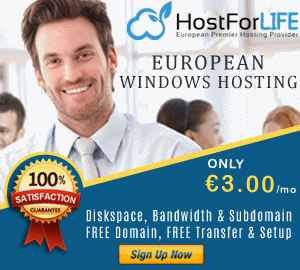
Database has a table named pictures with 3 columns:
1. ID Numeric Primary key with Identity Increment.
2. ImageName Varchar to store Name of picture.
3. Image column to store image in binary format.
After uploading and saving pictures in database, pics are displayed in GridView. And here is the HTML markup:
<form id="form1" runat="server">
<div>
<asp:TextBox ID="txtName" runat="server" Width="95px">
</asp:TextBox>
<asp:FileUpload ID="FileUpload1" runat="server"/>
<asp:Label ID="lblMessage" runat="server">
</asp:Label>
<asp:Button ID="btnUpload" runat="server"
OnClick="btnUpload_Click" Text="Upload"/>
</div>
</form>
Now, write the following code in Click Event of Upload Button:
protected void btnUpload_Click(object sender, EventArgs e)
{
string strImageName = txtName.Text.ToString();
if (FileUpload1.PostedFile != null &&
FileUpload1.PostedFile.FileName != "")
{
byte[] imageSize = new byte
[FileUpload1.PostedFile.ContentLength];
HttpPostedFile uploadedImage = FileUpload1.PostedFile;
uploadedImage.InputStream.Read
(imageSize, 0, (int)FileUpload1.PostedFile.ContentLength);
// Create SQL Connection
SqlConnection con = new SqlConnection();
con.ConnectionString = ConfigurationManager.ConnectionStrings
["ConnectionString"].ConnectionString;
// Create SQL Command
SqlCommand cmd = new SqlCommand();
cmd.CommandText = "INSERT INTO Images(ImageName,Image)" +
" VALUES (@ImageName,@Image)";
cmd.CommandType = CommandType.Text;
cmd.Connection = con;
SqlParameter ImageName = new SqlParameter
("@ImageName", SqlDbType.VarChar, 50);
ImageName.Value = strImageName.ToString();
cmd.Parameters.Add(ImageName);
SqlParameter UploadedImage = new SqlParameter
("@Image", SqlDbType.Image, imageSize.Length);
UploadedImage.Value = imageSize;
cmd.Parameters.Add(UploadedImage);
con.Open();
int result = cmd.ExecuteNonQuery();
con.Close();
if (result > 0)
lblMessage.Text = "File Uploaded";
GridView1.DataBind();
}
}
VB.NET Code
Protected Sub btnUpload_Click
(ByVal sender As Object, ByVal e As EventArgs)
Dim strImageName As String = txtName.Text.ToString()
If FileUpload1.PostedFile IsNot Nothing AndAlso
FileUpload1.PostedFile.FileName <> "" Then
Dim imageSize As Byte() = New Byte
(FileUpload1.PostedFile.ContentLength - 1) {}
Dim uploadedImage__1 As HttpPostedFile =
FileUpload1.PostedFile
uploadedImage__1.InputStream.Read(imageSize, 0,
CInt(FileUpload1.PostedFile.ContentLength))
' Create SQL Connection
Dim con As New SqlConnection()
con.ConnectionString =
ConfigurationManager.ConnectionStrings
("ConnectionString").ConnectionString
' Create SQL Command
Dim cmd As New SqlCommand()
cmd.CommandText = "INSERT INTO Images
(ImageName,Image) VALUES (@ImageName,@Image)"
cmd.CommandType = CommandType.Text
cmd.Connection = con
Dim ImageName As New SqlParameter
("@ImageName", SqlDbType.VarChar, 50)
ImageName.Value = strImageName.ToString()
cmd.Parameters.Add(ImageName)
Dim UploadedImage__2 As New SqlParameter
("@Image", SqlDbType.Image, imageSize.Length)
UploadedImage__2.Value = imageSize
cmd.Parameters.Add(UploadedImage__2)
con.Open()
Dim result As Integer = cmd.ExecuteNonQuery()
con.Close()
If result > 0 Then
lblMessage.Text = "File Uploaded"
End If
GridView1.DataBind()
End If
End Sub
HostForLIFE.eu SQL Server 2016 Hosting
HostForLIFE.eu is European Windows Hosting Provider which focuses on Windows Platform only. We deliver on-demand hosting solutions including Shared hosting, Reseller Hosting, Cloud Hosting, Dedicated Servers, and IT as a Service for companies of all sizes.
