
November 27, 2020 07:22 by
Peter
I have not found any direct way to call. So, we need to write our own logic inside a method provided by SignalR Library.
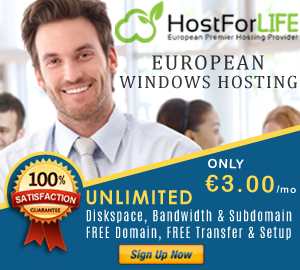
There is a Hub class provided by the SignalR library.
In this class, we have 2 methods.
OnConnectedAsync()
OnDisconnectedAsync(Exception exception)
So, the OnConnectedAsync() method will add user and OnDisconnectedAsyn will disconnect a user because when any client gets connected, this OnConnectedAsync method gets called.
In the same way, when any client gets disconnected, then the OnDisconnectedAsync method is called.
So, let us see it by example.
Step 1
Here, I am going to define a class SignalRHub and inherit the Hub class that provides virtual method and add the Context.ConnectionId: It is a unique id generated by the SignalR HubCallerContext class.
public class SignalRHub : Hub
{
public override Task OnConnected()
{
ConnectedUser.Ids.Add(Context.ConnectionId);
return base.OnConnected();
}
public override Task OnDisconnected()
{
ConnectedUser.Ids.Remove(Context.ConnectionId);
return base.OnDisconnected();
}
}
Step 2
In this step, we need to define our class ConnectedUser with property Id that is used to Add/Remove when any client gets connected or disconnected.
Let us see this with an example.
public static class ConnectedUser
{
public static List<string> Ids = new List<string>();
}
Now, you will get the result of currently connected client using ConnectedUser.Ids.Count.
As you see, here I am using a static class that will work fine when you have only one server, but when you will work on multiple servers, then it will not work as expected. In this case, you could use a cache server like Redis cache, SQL cache.